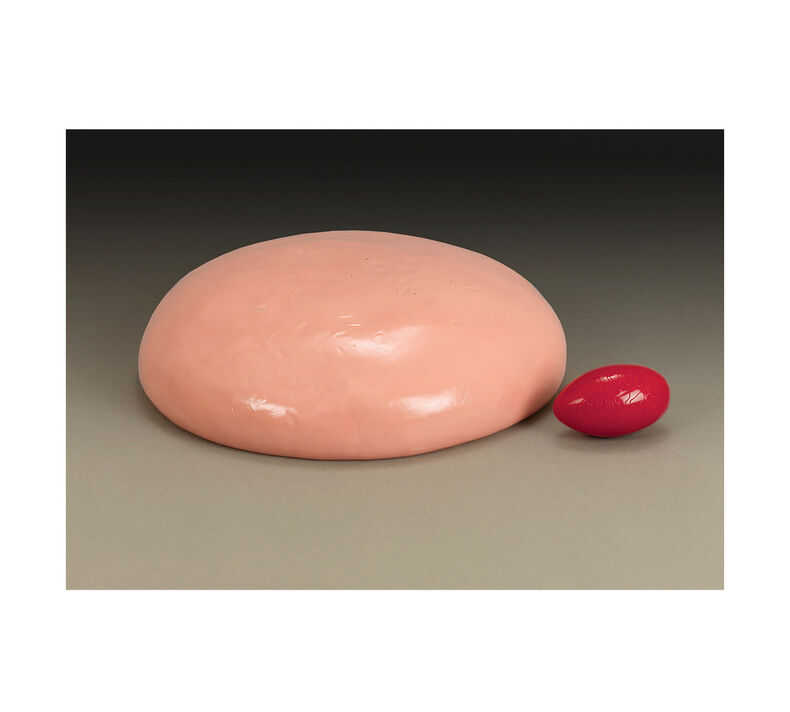
This blog is a little silly. It explains how to draw the Corona Virus using Python and turtle graphics.
What's included:
1) How to install Python
2) Short explanation of Python Turtle Graphics
3) Draw the Corona Virus in three easy steps (working Python programs)
Enjoy!
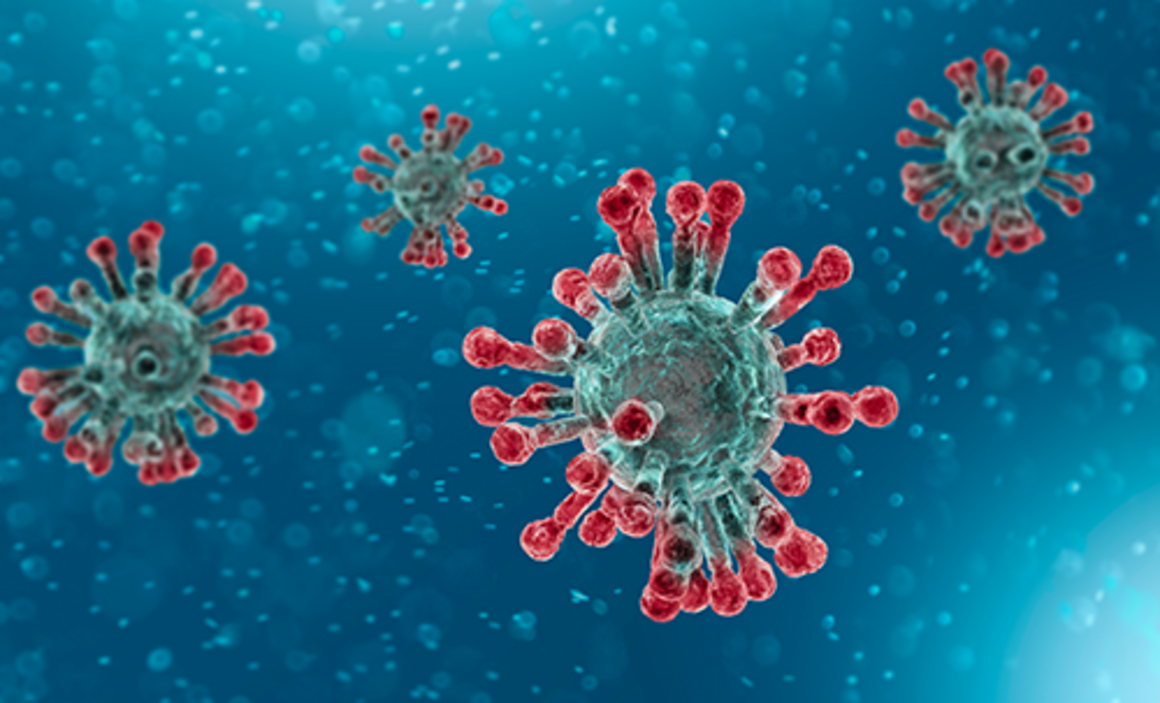